Full Stack Web Development
Using Angular, NodeJS, MongoDB, Java Spring Boot
Learn in-demand Modern Web DevelopmentSKILLS
Amplify your Career Opportunities
The Full-Stack Web Development course covers both front-end and back-end development, focusing on Angular for client-side and Java Spring Boot and Node.js for server-side.
Students learn to create robust web applications, integrating components, services, routing, and state management in Angular.
The course includes building RESTful APIs with Spring Boot and Express.js, along with database integration using MongoDB and MySQL.
Real-world projects such as e-commerce and social media platforms provide practical experience. By the end, students will develop a full-stack application, showcasing their expertise in modern web development technologies.
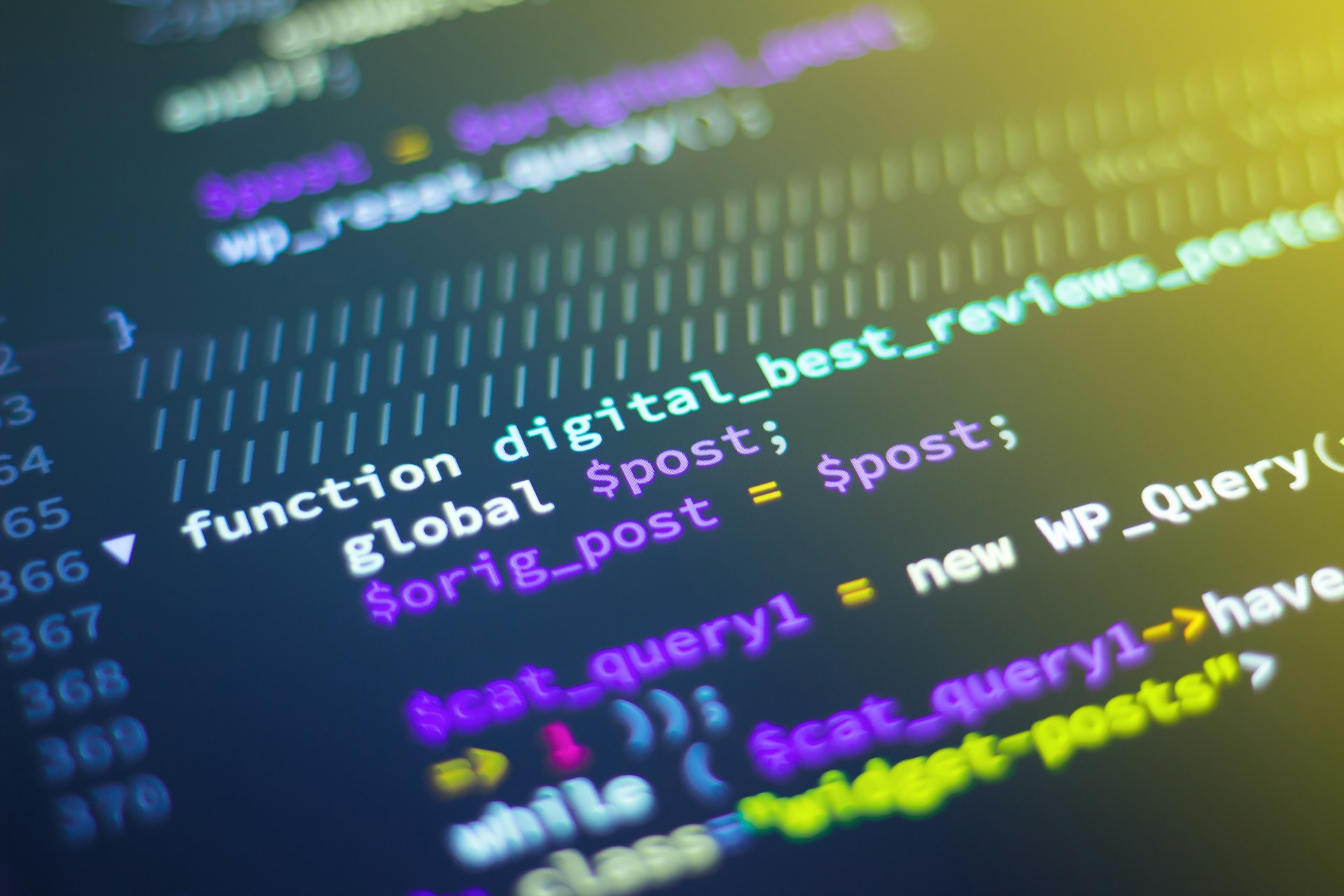
Development Roles
A full-stack web developer working with Angular, Node.js, MongoDB, and Java Spring typically has a diverse set of responsibilities, covering both the front-end and back-end aspects of web application development.
Our course covers these roles extensively
Front-End Development (Angular)
Design and Implement UI Components: Create reusable and modular UI components using Angular.
Responsive Design: Ensure the application is responsive and works well on various devices and screen sizes.
State Management: Manage application state using services and libraries like NgRx.
Back-End Development (Node.js)
RESTful APIs: Design and implement RESTful APIs using Express.js or NestJS.
GraphQL APIs: Develop GraphQL APIs if required, using frameworks like Apollo Server.
Back-End Development (Java Spring)
Spring Boot: Develop back-end services using Spring Boot for rapid development and deployment.
Spring MVC: Implement RESTful endpoints using Spring MVC.
Database Management (MongoDB)
Data Modeling: Design and optimize MongoDB schemas for efficient data storage and retrieval.
Indexes: Create indexes to improve query performance.
Course Outline
Download course here
Course Modules
Module 1: Introduction to Front-End and Back-End Development
- Overview of Web Development
- Client-Side vs. Server-Side Development
- Introduction to Angular, Java Spring Boot, and Node.js
- Setting Up Development Environment
Part 1: Front-End Development with Angular
Module 2: Introduction to Angular
- Introduction to Single Page Applications (SPA)
- Angular Architecture and Components
- Installing Angular CLI
- Creating and Running an Angular Application
Module 3: Angular Components and Templates
- Creating and Using Components
- Data Binding (Interpolation, Property, Event, and Two-Way Binding)
- Directives (Structural and Attribute)
- Angular Template Syntax
Module 4: Angular Services and Dependency Injection
- Creating and Using Services
- Dependency Injection in Angular
- HTTPClient for Making API Calls
- Handling Data with Observables and RxJS
Module 5: Angular Routing and Navigation
- Setting Up Angular Router
- Defining Routes and Navigation
- Route Guards and Lazy Loading
- Child Routes and Nested Navigation
Module 6: Forms in Angular
- Template-Driven Forms
- Reactive Forms
- Form Validation and Custom Validators
- Handling Form Data
Module 7: State Management in Angular
- Introduction to State Management
- Using Services for State Management
- Introduction to NgRx for State Management
- Implementing Redux Pattern in Angular
Module 8: Angular Modules and Advanced Topics
- Angular Modules and Lazy Loading
- Using Angular Material for UI Components
- Performance Optimization Techniques
- Unit Testing and End-to-End Testing in Angular
Part 2: Back-End Development with Java Spring Boot
Module 9: Introduction to Java Spring Boot
- Overview of Spring Framework
- Setting Up Spring Boot Project
- Understanding Spring Boot Architecture
- Creating a Basic Spring Boot Application
Module 10: Spring Boot RESTful Web Services
- Creating RESTful APIs with Spring Boot
- Handling HTTP Methods (GET, POST, PUT, DELETE)
- Data Validation and Exception Handling
- Consuming APIs with Angular
Module 11: Spring Data JPA and Database Integration
- Introduction to Spring Data JPA
- Setting Up Database Connection
- CRUD Operations with JPA Repositories
- Using Hibernate with Spring Boot
Module 12: Security in Spring Boot
- Introduction to Spring Security
- Implementing Authentication and Authorization
- JWT (JSON Web Token) for Secure APIs
- Security Best Practices
Module 13: Advanced Spring Boot Features
- Spring Boot Actuator for Monitoring
- Scheduling Tasks with Spring Boot
- Sending Emails with Spring Boot
- Integrating Third-Party APIs
Part 3: Back-End Development with Node.js
Module 14: Introduction to Node.js
- Overview of Node.js and its Features
- Setting Up Node.js Environment
- Node.js Architecture and Event Loop
- Creating a Basic Node.js Application
Module 15: Building RESTful APIs with Express.js
- Introduction to Express.js
- Setting Up Express.js Project
- Creating RESTful Endpoints
- Middleware and Error Handling
Module 16: Database Integration with Node.js
- Connecting to MongoDB using Mongoose
- Creating Schemas and Models
- CRUD Operations with Mongoose
- Using MySQL with Node.js
Module 17: Authentication and Authorization in Node.js
- User Authentication with Passport.js
- Implementing JWT for Authentication
- Role-Based Access Control
- Securing Node.js APIs
Module 18: Advanced Node.js Features
- Real-Time Communication with Socket.io
- Building GraphQL APIs with Node.js
- File Uploads and Data Streaming
- Performance Optimization in Node.js
Part 4: Full-Stack Development Projects
Module 19: Full-Stack Development Workflow
- Setting Up Full-Stack Project
- Connecting Angular Front-End with Spring Boot Back-End
- Connecting Angular Front-End with Node.js Back-End
- Environment Configuration and Deployment
Module 20: Full-Stack Project 1: E-commerce Application
- Building Front-End with Angular
- Building Back-End with Spring Boot
- Implementing Shopping Cart and Payment Gateway
- Deployment and Maintenance
Module 21: Full-Stack Project 2: Social Media Platform
- Building Front-End with Angular
- Building Back-End with Node.js
- Implementing Real-Time Features with Socket.io
- Deployment and Maintenance
Capstone Project
- Real-World Full-Stack Application Development
- End-to-End Integration of Angular, Spring Boot, and Node.js
- Project Planning and Execution
- Presentation and Defense of the Project
Assessment and Certification
- Quizzes and Exams
- Practical Lab Assessments
- Final Project Evaluation
- Certification of Completion
Make the first step by discussing with our course coordinator
Course Delivery
Live & On-line Learning